The concept of manual testing can be applied without tools because what we need is a quality analyst and an application with a complete environment, that is, the backend services responding to our application.
E2E tests are performed by specific tools that simulate user behavior. Up to version 14, Angular already had a built-in tool called Protractor, but the Angular team no longer recommends it because there are more modern, faster alternatives. In this chapter, we are going to use Cypress for this purpose.
Finally, unit tests are performed on the methods of our services and components, verifying their behavior.
In the Angular toolbox, we have two tools for creating and running these tests: Jasmine and Karma. These tools are installed by default when we start a new project.
Jasmine is a testing framework that has several checking functions, in addition to providing the ability to change the functionality of a method or class at runtime with an element called a spy. For the execution of unit tests, the Karma tool is used, which has the characteristic of running tests in a browser, giving the team the ability to analyze the behavior of the application in different types of environments. Although rare nowadays, we may have some bugs depending on the browser it runs on.
To use these two tools, we don’t need any configuration of our project; we just need to execute the following command in the command line of our operating system:
ng test
Once we execute the preceding command, we get a compilation error. This happens because, until then, Angular only compiled the files of our components and ignored the test files because they will not be deployed to users in the final version.
Running the test, we notice that we have an error in the diary.resolver.spec.ts test file, so let’s make a correction:
describe(‘diaryResolver’, () => {
const executeResolver: ResolveFn<
ExerciseSetList
> = (…resolverParameters) =>
TestBed.runInInjectionContext(() => diaryResolver(…resolverParameters));
beforeEach(() => {
TestBed.configureTestingModule({});
});
it(‘should be created’, () => {
expect(executeResolver).toBeTruthy();
});
});
The test generated by the Angular CLI contains all of Jasmine’s boilerplate. In the describe function, we define our test case, which is a test group that we will create.
This function has, in the first parameter, a string that represents the name of the test case and will even identify it in reports.
In the second parameter, we have the function where we will have the preparation and the tests. Here, we made a correction because the resolver we want to test returns an object of type ExerciseSetList and not a Boolean as it was before.
On the next line, we have the TestBed class, which is the most fundamental element of Angular tests.
This framework class has the function of preparing the Angular execution environment for the tests to run. We will see in the following sections its use in different situations.
The beforeEach function has the objective of performing some common action before executing the tests.
Finally, the it function is where we will create the tests. Inside a describe function, we can have numerous functions of the it type.
If we run Karma again, the browser will open, and we can follow the execution of the tests:
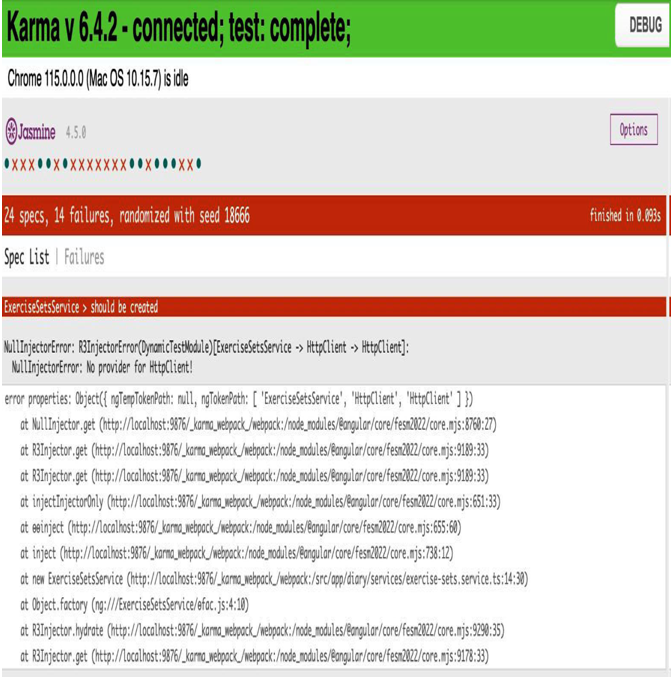
Figure 10.2 – Execution of tests by Karma
As this project already had the tests when we created the elements of our application, we have some broken tests that we will correct in the next sections, but the important thing now is for us to understand how to run the unit tests of our application.
In the next section, we’ll learn how to create tests for our project’s services.