One of the best practices in a software project, be it a frontend or backend project, is testing. After all, if you and your team don’t rigorously test your system, the people who will inevitably test the system and find possible bugs are the users, and we don’t want that.
For this reason, it is no wonder that the Angular team has, since the first versions of the framework, been concerned with creating and integrating automated testing tools.
We can notice this with the fact that, by default, the Angular CLI always generates, together with the component, its test files as if saying, “Hey, buddy, don’t forget the unit test!”
In this chapter, we will explore this topic by covering the following:
- What to test
- Service tests
- Understanding TestBed
- Component testing
- E2E tests with Cypress
At the end of the chapter, you will be able to create tests for your components and service, improving the quality of your delivery and your team.
Technical requirements
To follow the instructions in this chapter, you’ll need the following:
- Visual Studio Code (https://code.visualstudio.com/Download)
- Node.js 18 or higher (https://nodejs.org/en/download/)
The code files for this chapter are available at https://github.com/PacktPublishing/Angular-Design-Patterns-and-Best-Practices/tree/main/ch10.
During the study of this chapter, remember to run the backend of the application found in the gym-diary-backend folder with the npm start command.
What to test
Within a software project, we can do several types of tests to ensure the quality of the product. In this discipline, it is very common to categorize tests using a pyramid.
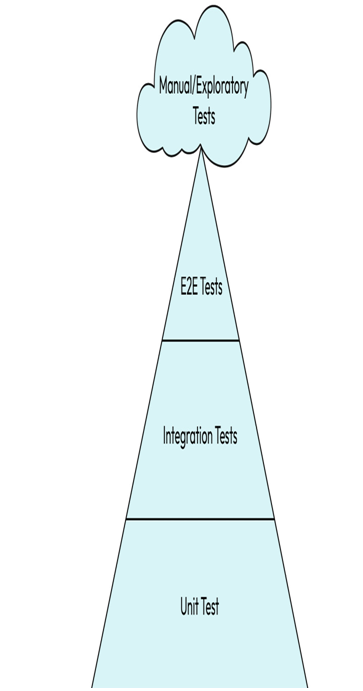
Figure 10.1 – Test pyramid
At the base of the pyramid, we have unit tests, whose objective is to verify the quality of the smallest elements within a software project, such as functions or methods of a class. Due to their narrow scope and atomic nature, they are quickly executed by tools and should ideally make up the majority of an application’s tests.
In the middle layer, we have integration tests, which are focused on verifying how the project components interact with each other, being able, for example, to test an API through an HTTP request. Because these tests use more elements and need certain environmental requirements, they are less performant and have a higher execution cost, which is why we see them in smaller quantities compared to unit tests.
At the top of the pyramid, we have end-to-end tests (E2E tests), which validate the system from the user’s point of view, emulating their actions and behaviors. These tests require an almost complete environment, including a database and servers. In addition, they are slower and therefore there are fewer of them compared to the previous ones.
Finally, we have manual and exploratory tests, which are tests performed by quality analysts. Ideally, these tests will serve as a basis for the creation of E2E tests, mainly on new features. As they are run by humans, they are the most expensive, but they are the best for discovering new bugs in new features.
It is important to highlight that no test is better or more important than another. Here, we have the classification by volume of test executions in a period of time. You and your team must identify which tests to prioritize based on the capacity and resources available for your project. These types of tests can be applied to any type of software project, but you must be wondering how we fit this concept into an Angular project.