Reactive forms use a declarative and explicit approach to creating and manipulating form data. Let’s put this concept into practice by creating a new form for our project.
First, on the command line, let’s use the Angular CLI to generate the new component:
ng g c diary/new-entry-form-reactive
In the same way as we did with the template-driven form, let’s add this new component to the DiaryRoutingModule routing module:
import { NewEntryFormReactiveComponent } from ‘./new-entry-form-reactive/new-entry-form-reactive.component’;
const routes: Routes = [
{
path: ”,
component: DiaryComponent,
},
{
path: ‘new-template’,
component: NewEntryFormTemplateComponent,
},
{
path: ‘new-reactive’,
component: NewEntryFormReactiveComponent,
},
];
In the DiaryModule module, we need to add the ReactiveFormsModule module responsible for all the functionality that Angular makes available to us for this type of form:
@NgModule({
declarations: [
.
.
.
],
imports: [
.
.
.
ReactiveFormsModule
,
],
})
To finalize the component’s route, let’s change the main screen of our application, replacing the route that the New Entry button will call:
addExercise(newSet: ExerciseSet) {
this.router.navigate([‘/home/new-reactive’]);
}
We will now start creating the reactive form. First, let’s configure the component elements in the new-entry-form-reactive.component.ts TypeScript file:
export class NewEntryFormReactiveComponent implements OnInit {
public entryForm!: FormGroup;
private formBuilder = inject(FormBuilder);
ngOnInit() {
this.entryForm = this.formBuilder.group({
date: [”],
exercise: [”],
sets: [”],
reps: [”],
});
}
}
Note that the first attribute is entryForm of type FormGroup. It will represent our form—not just the data model, but the whole form—as validations, field structure, and so on.
Then, we inject the FormBuilder service responsible for assembling the entryForm object. Note the name of the service that Angular uses from the Builder design pattern, which has the objective of creating complex objects, such as a reactive form.
To initialize the entryForm attribute, we’ll use the onInit component lifecycle hook. Here, we’ll use the group method to define the form’s data model. This method receives the object, and each attribute receives an array that contains the characteristics of that attribute in the form. The first element of the array is the initial value of the attribute.
In the component’s template, we will create the structure of the form, which, in relation to the template-driven form example, is very similar:
<div class=”flex h-screen items-center justify-center bg-gray-200″>
<form
[formGroup]=”entryForm”
>
<input
type=”date”
id=”date”
name=”date”
formControlName=”date”
/>
<input
type=”text”
id=”exercise”
name=”exercise”
formControlName=”exercise”
/>
<input
type=”number”
id=”sets”
name=”sets”
formControlName=”sets”
/>
<input
type=”number”
id=”reps”
name=”reps”
formControlName=”reps”
/>
<button type=”submit”>
Add Entry
</button>
{{ entryForm.value | json }}
The first difference is the use of the formGroup attribute to associate the template with the object we created earlier. To associate each template field to the FormGroup attribute, we use the formControlName element.
To debug the data model, we are also using the JSON pipe, but note that to get the data model filled in by the user, we use the value attribute of the entryForm object. Finally, we will complement the form with functionality and record the input using the project’s API.
The next step is to change the component:
export class NewEntryFormReactiveComponent implements OnInit {
.
.
.
private exerciseSetsService = inject(ExerciseSetsService);
private router = inject(Router);
.
.
.
newEntry() {
const newEntry = { …this.entryForm.value };
this.exerciseSetsService
.addNewItem(newEntry)
.subscribe((entry) => this.router.navigate([‘/home’]));
}
}
Here, we inject the consumer services of the ExerciseSetsService API and the Angular route service router.
In the newEntry method, as in the previous example, we capture the data that the user typed. However, in the reactive form, it is in the value attribute, and we send this attribute to the API using the service.
Running the project, we can see that the interface works like its counterpart written for the template-driven form.
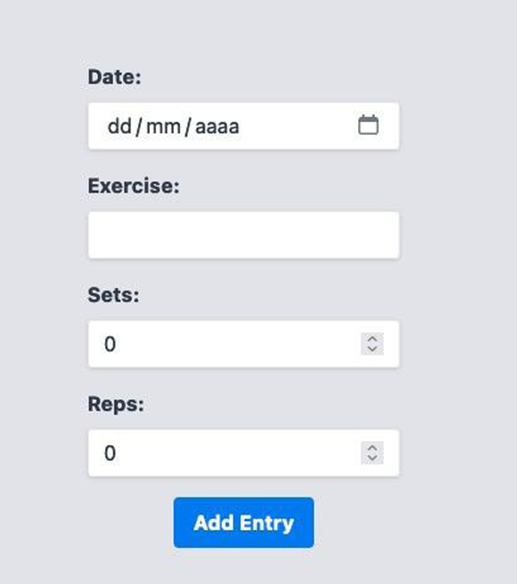
Figure 6.2 – Gym Diary Form UI using a reactive -form
You may be wondering, what is the advantage of using the reactive form and why is it recommended by the Angular community and team? Next, we’ll see how to use the form’s built-in validations and how to integrate them into our reactive form.