In addition to the loading screen to inform the user that the system is looking for the information they want, it is important to notify the user after processing an item. We can handle this notification directly from the service or component, but we can also implement it generically and implicitly using interceptors.
We will refactor our application to add this treatment. But first, let’s install a library to show the toaster component on the screen with an animation. In the command line of our operating system, we will use the following command in the main folder of our frontend project:
npm install ngx-toastr
In order for the package to work, we need to add our CSS to our project by editing the angular.json file:
“build”: {
“assets”: [
“src/favicon.ico”,
“src/assets”
],
“styles”: [“src/styles.css”,
“node_modules/ngx-toastr/toastr.css”
],
},
For the toaster animations to work, we need to change the AppModule module:
imports: [
BrowserAnimationsModule,
AppRoutingModule,
HttpClientModule,
ToastrModule.forRoot(),
],
In the main module of our application, we are adding the ToastrModule module from the library and changing BrowserModule to BrowserAnimationsModule, which adds Angular animation services used by the library.
With the new package configured, we can proceed with creating the new interceptor using the Angular CLI:
ng interceptor notification/notification
With the interceptor created, we will change the file with the treatment for the notification:
import { ToastrService } from ‘ngx-toastr’;
@Injectable()
export class NotificationInterceptor implements HttpInterceptor {
private toaster = inject(ToastrService);
intercept(
request: HttpRequest<unknown>,
next: HttpHandler
): Observable<HttpEvent<unknown>> {
return next.handle(request).pipe(
tap((event: HttpEvent<any>) => {
if (event instanceof HttpResponse && event.status === 201) {
this.toaster.success(‘Item Created!’);
}
})
);
}
}
As in the Creating a loader section, we are using the fact that the request is treated as a flow to use RxJS and its observables to verify the request’s characteristics. We are using the tap operator, which aims to perform side effects on the request without changing it.
This operator will execute an anonymous function that will check the HTTP event, which brings us to an interesting point. As we are interested in the return of the request, we only select the event of type HttpResponse and the event code is 201-Created.
When we develop an interceptor, we have to remember that it is called in the request and the response, so it is important to use conditionals to execute what we need when we need it.
The last point we need to configure is the main AppModule module:
providers: [
{
provide: HTTP_INTERCEPTORS,
useClass: NotificationInterceptor,
multi: true,
},
]
Running our project and creating an entry, we notice that the toast appears on the screen with the configured message.
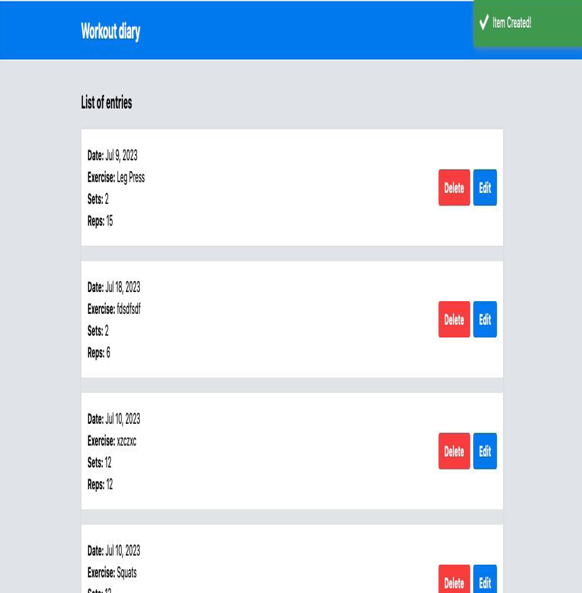
Figure 8.4 – Success notification
Another use for interceptors is to instrument our application to measure performance and stability, which we’ll learn about in the next section.