The RxJS library has an extensive number of operators that can help simplify your code and handle corner cases of asynchrony and even performance.
You don’t need to memorize all the operators and the ones we’ve seen so far will help you with the most common cases.
The library documentation has a Decision Tree page, and we’ll learn how to navigate that.
Enter the site (https://rxjs.dev/operator-decision-tree) and, here, we will navigate to an operator that we have already studied to exemplify the use of this tool.
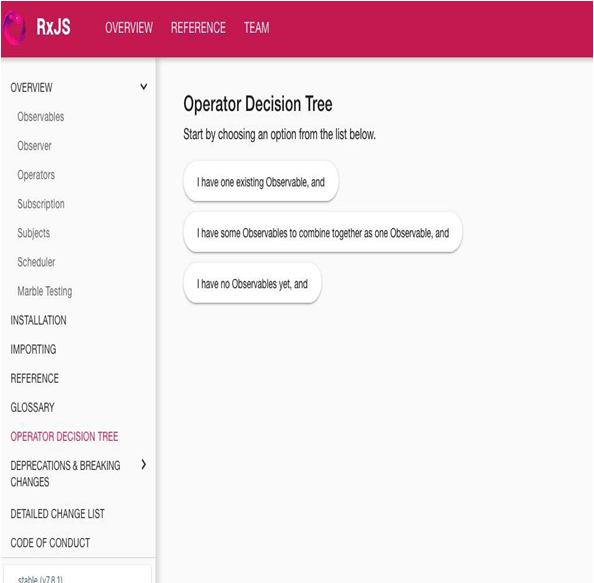
Figure 9.2 – Operator Decision Tree
Let’s go back to our form example. We need to fetch the exercise information from what the user’s typing – let’s assume that we don’t know which operator to choose.
We already have an observable, which is the valueChanges event in the Angular form, so on the first screen, we will choose the I have one existing Observable, and option.
The request to our API is represented by an observable, so on the next screen, we will choose the I want to start a new Observable for each value option.
As we want to make a new request for each letter the user types, we want to change one stream for another, so on the next screen, we’ll choose and cancel the previous nested Observable when a new value arrives.
The exercise search depends on the value that is in the Angular form, so on the final page, we will choose where the nested Observable is calculated for each value.
Confirming the selection, the decision tree indicates that the correct operator for this situation is the switchMap operator that we are using!
Another thing we need to understand in the RxJS documentation is the marble graph. For this, let’s take as an example another operator that we studied in the chapter, the map operator here: https://rxjs.dev/api/index/function/map.
In addition to the textual explanation, we have the following figure:
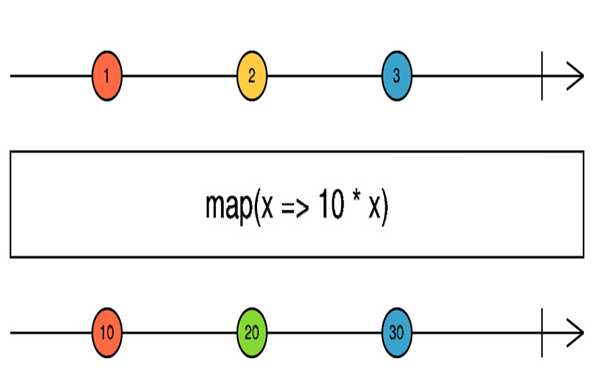
Figure 9.3 – Map operator marble graph (source: https://rxjs.dev/api/index/function/map, MIT license)
As we learned at the beginning of this chapter, RxJS works on information flows, where operators have the function of handling information.
The graph that illustrates this flow uses arrows to represent the passage of time and marbles to represent values.
In the documentation here, then, we see that the map operator takes each value emitted and, based on a function, results in a flow with the values transformed by it.
These values are exchanged one by one as soon as they are issued so, in the graph, we can see that the positions of the marbles are same.
This understanding is fundamental to understanding other more complex operators in the library.
Summary
In this chapter, we explored the RxJS library and its basic elements, observables.
We learned what an observable is and how it differs from a promise or a function. With that knowledge, we refactored our project to handle data with the map operator, abstracting the implementation details of the component that will consume the service. We also learned about Angular’s async pipe and how it simplifies the management of subscription to an observable, leaving this task to the framework itself to manage.
Finally, we created a typeahead search field using RxJS to search for exercises based on the user’s typing event, using operators in order to optimize HTTP calls from our frontend. In the next chapter, we will explore the possibilities of the automated tests that we can do in our Angular application.