In our current project, if the user enters a path that does not have a mapped route, they will be faced with a blank screen. This is not a good user experience (UX) practice; ideally, we need to handle this error by presenting an error page for it to be redirected to the correct page.
First, let’s create the component using the Angular CLI:
ng generate component ErrorPage
Here, we are creating the component directly in AppModule because we want to give this treatment to our entire system and not to a specific functional module.
Let’s create the template for this component with the error message:
<div class=”flex h-screen flex-col items-center justify-center”>
<h1 class=”mb-4 text-6xl font-bold text-red-500″>Oops!</h1>
<h2 class=”mb-2 text-3xl font-bold text-gray-800″>Looks like you’re lost!</h2>
<p class=”mb-6 text-gray-600″>
We couldn’t find the page you’re looking for.
</p>
<p class=”text-gray-600″>
But don’t worry!
Go back to the Gym Diary and continue your progress!
</p>
<a
routerLink=”/home”
class=”mt-4 rounded bg-blue-500 px-4 py-2 font-bold text-white hover:bg-blue-600″
>
Go back to the Gym Diary
</a>
</div>
Note that we have the link to the home page as a call to action for the user to return to the home page.
The next step is to update the AppRoutingModule routes file:
import { ErrorPageComponent } from ‘./error-page/error-page.component’;
const routes: Routes = [
{ path: ”, pathMatch: ‘full’, redirectTo: ‘home’ },
{
path: ‘home’,
loadChildren: () =>
import(‘./home/home.module’).then((file) => file.HomeModule),
},
{ path: ‘error’, component: ErrorPageComponent },
{ path: ‘**’, redirectTo: ‘/error’ },
];
At this point, Angular will do its job. Just by defining the error page route and then creating another entry in the array, we have defined the ‘**’ path and redirected it to the error route.
When we run our project, if the user enters an incorrect page, the following message will be displayed:
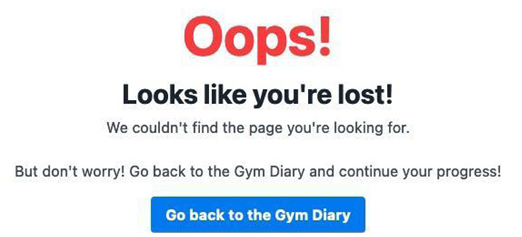
Figure 7.2 – Incorrect route error page
Another point that we can improve in our application is the title of the page in the Browser tab.
For this, we can once again use Angular’s routing mechanisms. In DiaryRoutingModule, we need to change the following code snippet:
const routes: Routes = [
{
path: ”,
component: DiaryComponent,
title: ‘Diary’,
},
{
path: ‘new-template’,
component: NewEntryFormTemplateComponent,
},
{
path: ‘new-reactive’,
component: NewEntryFormReactiveComponent,
title: ‘Entry Form’,
},
];
To change the title, we just need to inform the title property in the route definition. Another approach that is possible (but longer) is to use Angular’s Title service.
Let’s exemplify this in the NewEntryFormTemplateComponent component:
import { Title } from ‘@angular/platform-browser’;
export class NewEntryFormTemplateComponent implements OnInit {
private titleService = inject(Title);
ngOnInit(): void {
this.titleService.setTitle(‘Template Form’);
}
}
After injecting the Title service, we are using it in the OnInit lifecycle hook. Although the route approach is much simpler and more intuitive, the Title service can be used if the title can change dynamically.
We will now learn how to pass information from one route to another in the next section.