A good UX practice is to validate the information that users enter in the form as soon as it leaves the filled field. This minimizes user frustration while improving the information that will be sent to the backend.
Using reactive forms, we can use utility classes created by the Angular team to add validations that are commonly used in forms. Let’s improve our project, first in the NewEntryFormReactiveComponent component:
.
.
.
import { FormBuilder, FormGroup, Validators } from ‘@angular/forms’;
.
.
.
export class NewEntryFormReactiveComponent implements OnInit {
.
.
.
ngOnInit() {
this.entryForm = this.formBuilder.group({
date: [”, Validators.required],
exercise: [”, Validators.required],
sets: [”, [Validators.required, Validators.min(0)]],
reps: [”, [Validators.required, Validators.min(0)]],
});
}
newEntry() {
if (this.entryForm.valid) {
const newEntry = { …this.entryForm.value };
this.exerciseSetsService
.addNewItem(newEntry)
.subscribe((entry) => this.router.navigate([‘/home’]));
}
}
}
In the preceding example, we are importing the Validators package from Angular that will provide the utility class for the basic validations of our report. In the ngOnInit method where we create the reactive form object, the validations are in the second position of the array that defines the form’s fields.
We use the required validation in all fields of the form, and in the sets and reps fields, we add another validation to guarantee that the number is positive. To add more than one validation, we can add another array with the validations.
Another change we made to our component is that it now checks whether the form is valid before starting the interaction with the backend. We do this by checking the valid attribute of the object. Angular automatically updates this field as the user enters data.
In the template file, let’s add the error messages for the user:
<div
*ngIf=”entryForm.get(‘date’)?.invalid && entryForm.get(‘date’)?.touched”
class=”mt-1 text-red-500″
>
Date is required.
</div>
<div
*ngIf=”
entryForm.get(‘exercise’)?.invalid &&
entryForm.get(‘exercise’)?.touched
“
class=”mt-1 text-red-500″
>
Exercise is required.
</div>
.
.
.
<div
*ngIf=”entryForm.get(‘sets’)?.invalid && entryForm.get(‘sets’)?.touched”
class=”mt-1 text-red-500″
>
Sets is required and must be a positive number.
</div>
<div
*ngIf=”entryForm.get(‘reps’)?.invalid && entryForm.get(‘reps’)?.touched”
class=”mt-1 text-red-500″
>
Reps is required and must be a positive number.
</div>
<button
type=”submit”
[disabled]=”entryForm.invalid”
[class.opacity-50]=”entryForm.invalid”
>
Add Entry
</button>
To show validation in the template, we use div elements with the message we want. To decide whether or not the message will appear, we use the ngIf directive, checking the status of the field.
For this, we first get the field using the GET method and check the following two properties:
- The invalid property checks whether the field is invalid according to what was configured in the component.
- The touched property checks whether the user has accessed the field. It is recommended not to show all the validations when the interface is loaded.
In addition to the validations in each field, to improve usability, we changed the Submission button by disabling it while the form was invalid and applying the CSS to make it clear to the user.
Running the project, we can see the validations accessing all fields without filling any field.
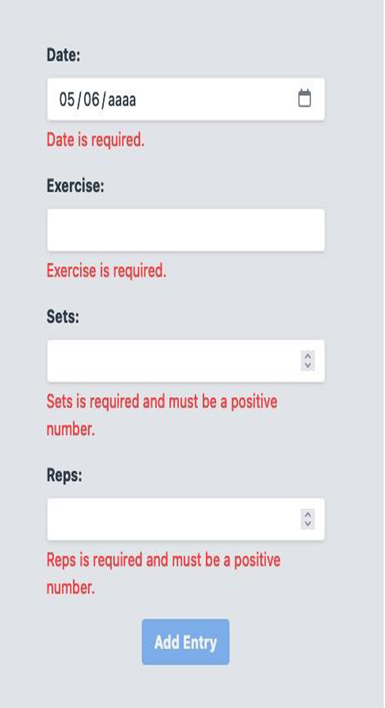
Figure 6.3 – Gym Diary Form UI validations
We’ve learned how to use Angular’s utility classes to perform validation, so let’s explore how we can create our own custom validations.